- Tcl Program To Calculate The Time Difference Between Two Times Square
- Tcl Program To Calculate The Time Difference Between Two Times Crossword
- Tcl Program To Calculate The Time Difference Between Two Times Two
In the first case you should obtain the current time before and after the event, and calculate the interval between them (for example, with the aqDateTime.TimeInterval method). In the second case you should create an instance of StopWatch object, start the time count using the StopWatch.Start method, execute the desired operation and then stop. In this program, user is asked to enter two time periods and these two periods are stored in structure variables startTime and stopTime respectively. Then, the function differenceBetweenTimePeriod calculates the difference between the time periods and the result is displayed in main function without returning it (Using call by reference. C Program to Calculate Difference Between Two Time Periods In this example, you will learn to calculate the difference between two time periods using a user-defined function. To understand this example, you should have the knowledge of the following C programming topics. The environment variable TCLTZ. The environment variable TZ. On Windows systems, the time zone settings from the Control Panel. If none of these is present, the C localtime and mktime functions are used to attempt to convert times between local and Greenwich. On 32-bit systems, this approach is likely to have bugs, particularly for times that.
This is a simple guide on how to calculate the difference between two dates in PHP. Be sure to try out the examples below if you're new to the topic.
Our dates.
Let us say, for example, that we have two date strings and that they are formatted like so:
- 2013-03-01 19:12:45
- 2014-03-01 06:37:04
As you can see, there's about a year in the difference between them.
However, what if we want to calculate the exact number of seconds that have passed between date one and date two?
2 4 6 8 10 12 14 | $date1='2013-03-01 19:12:45'; $date1Timestamp=strtotime($date1); $difference=$date2Timestamp-$date1Timestamp; echo$difference; |
If you run the code above, you'll see that the answer is: 31490659. i.e. 31490659 seconds passed between '2013-03-01 19:12:45' and '2014-03-01 06:37:04'.
A basic drill down of what I did:
- I converted both of our date strings into a unix timestamp by using the built-in PHP function strtotime. A unix timestamp represents the number of seconds that have passed since 00:00 on the 1st of January, 1971. By converting them into Unix Time, I've converted the dates into a format that allows me to do some basic calculations.
- I then subtracted the older date from the newer date.
Remember: The older a date is, the smaller its corresponding timestamp will be! The jackbox party pack 4 for mac. Run the following example to see what I mean:
2 4 6 | $newerDate='2014-06-07'; echo$olderDate.' is '.strtotime($olderDate),' '; |
As you can see, '2014-06-07' has a much bigger timestamp, simply because more seconds have passed since the 1st of January, 1971 and the 7th of June, 2014.
Between then and now.
What if you need to find out how many seconds have passed since a given date?
2 4 6 8 10 12 | $then='2011-02-02 08:00:00'; $thenTimestamp=strtotime($then); //Get the difference in seconds. |
In the code above:
- I've used the function time to retrieve the current timestamp. i.e. Our current Unix Time.
- I then subtracted the older timestamp from our current timestamp.
If you continue to refresh the page, you'll see that the difference in seconds will continue to grow.
Minutes & Days…
In many cases, you'll want the number of days or minutes that have passed. Let's face it: Seconds are kind of useless to an end user.
To calculate the number of days that have passed:
2 4 6 8 10 12 14 16 18 | $then='2009-02-04'; //Convert it into a timestamp. $now=time(); //Calculate the difference. $days=floor($difference/(60*60*24)); echo$days; |
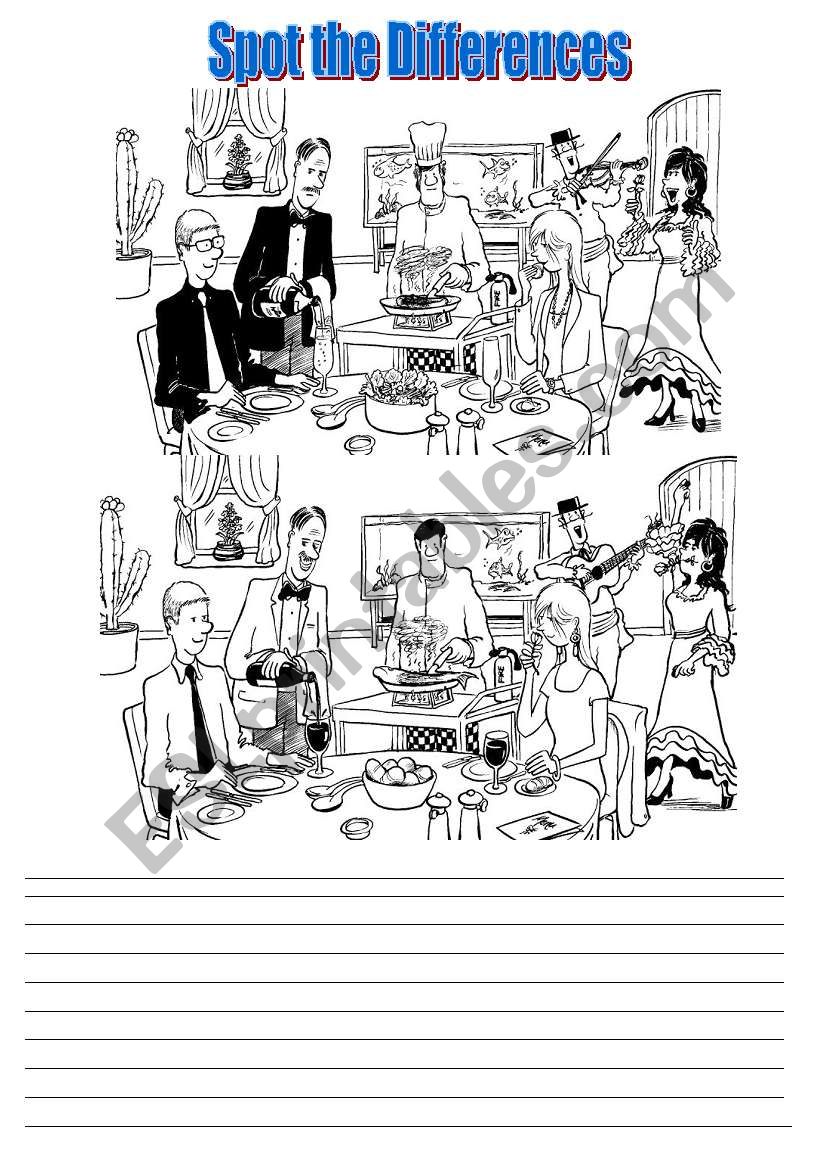
As you can see, I was able to convert seconds into days via some basic math:
- There are 60 seconds in a minute.
- There are 60 minutes in an hour.
- There are 24 hours in a day.
If you multiply the figures above, it will give you 86400, which is total the number of seconds that is in a given day. Divide the difference in seconds between our two dates by 86400 and you've got the number of days that have passed.
You can then use the floor function in order to round the result down. i.e. 2.9 days = 2 days.
To get the number of minutes, you can simply divide the difference in seconds by 60, like so:
Tcl Program To Calculate The Time Difference Between Two Times Square
2 4 6 8 10 12 14 16 18 | $then='2009-02-04'; //Convert it into a timestamp. $now=time(); //Calculate the difference. $minutes=floor($difference/60); echo$minutes; |
As you can see, it's pretty simple.
OOP
Once you've grasped the fundamentals of dealing with dates and timestamps in PHP, you should look into using the DateTime class, simply because it's cleaner and it provides you with an OO interface.
For example, if we want to get the difference in days using the DateTime class, we can do the following:
2 4 6 8 | $date2=newDateTime(); $diff=$date2->diff($date1)->format('%a'); echo$diff; |
Much better.
To transform the difference into a human-readable format that is comprised of years, months, days, hours and minutes:
2 4 6 8 10 12 14 | $then=newDateTime($then); $now=newDateTime(); $sinceThen=$then->diff($now); //Combined echo$sinceThen->m.' months have passed. '; echo$sinceThen->h.' hours have passed. '; |
As you can see, the DateTime class is pretty powerful once you figure out to use it!
Facebook Comments
Note
Office 365 ProPlus is being renamed to Microsoft 365 Apps for enterprise. For more information about this change, read this blog post.
Summary
This article describes how Microsoft Access stores the Date/Time data type. This article also describes why you may receive unexpected results when you calculate dates and times or compare dates and times.
This article describes the following topics:
- Store Date/Time data
- Format a Date/Time field
- Calculate time data
- Compare date data
- Compare time data
More Information
Store Date/Time data
Access stores the Date/Time data type as a double-precision, floating-point number up to 15 decimal places. The integer part of the double-precision number represents the date. The decimal portion represents the time.
Valid date values range from -657,434 (January 1, 100 A.D.) to 2,958,465 (December 31, 9999 A.D.). A date value of 0 represents December 30, 1899. Access stores dates before December 30, 1899 as negative numbers.
Valid time values range from .0 (00:00:00) to .99999 (23:59:59). The numeric value represents a fraction of one day. You can convert the numeric value to hours, to minutes, and to seconds by multiplying the numeric value by 24.
The following table shows how Access stores Date/Time values:
Double number | Date portion | Actual date | Time portion | Actual time |
---|---|---|---|---|
1.0 | 1 | December 31, 1899 | .0 | 12:00:00 A.M. |
2.5 | 2 | January 1, 1900 | .5 | 12:00:00 P.M. |
27468.96875 | 27468 | March 15, 1975 | .96875 | 11:15:00 P.M. |
36836.125 | 36836 | November 6, 2000 | .125 | 3:00:00 A.M. |
To view how Access stores Date/Time values as numbers, type the following commands in the Immediate window, press ENTER, and then notice the results:
?CDbl(#5/18/1999 14:00:00#)
Result equals: 36298.5833333333
?CDbl(#12/14/1849 17:32:00#)
Result equals: -18278.7305555556
To view the date and the time of numeric values, type the following commands in the Immediate window, press ENTER, and then notice the results:
?CVDate(1.375)
Result equals: 12/31/1899 9:00:00 AM Old time slot machines.
?CVDate(-304398.575)
Result equals: 8/1/1066 1:48:00 PM
Format a Date/Time field
You can format a Date/Time value to display a date, a time, or both. When you use a date-only format, Access stores a value of 0 for the time portion. When you use a time-only format, Access stores a value of 0 for the date portion.
The following table shows how Access stores Date/Time values. The following table also shows how you can display those values by using different formats:
|Stored value (double number)| Default format (General Date)| Custom format(mm/dd/yyyy hh:nn:ss A.M./P.M.)||-------------------|---------------------|-----------------------------||36295.0| 5/15/99| 05/15/1999 12:00:00 AM||0.546527777777778| 1:07 PM| 12/30/1899 01:07:00 PM||36232.9375| 3/13/99| 10:30PM 03/13/1999 10:30:00 PM|
Tcl Program To Calculate The Time Difference Between Two Times Crossword
Note How to open a .package file. The default format for a Date/Time value is General Date. If a value is date-only, no time appears. If the value is time-only, no date appears.
Calculate time data
Because a time value is stored as a fraction of a 24-hour day, you may receive incorrect formatting results when you calculate time intervals greater than 24 hours. To work around this behavior, you can create a user-defined function to make sure that time intervals are formatted correctly.
Microsoft provides programming examples for illustration only, without warranty either expressed or implied. This includes, but is not limited to, the implied warranties of merchantability or fitness for a particular purpose. This article assumes that you are familiar with the programming language that is being demonstrated and with the tools that are used to create and to debug procedures. Microsoft support engineers can help explain the functionality of a particular procedure, but they will not modify these examples to provide added functionality or construct procedures to meet your specific requirements. To correctly calculate and to format time intervals, follow these steps:
Create a module, and then type the following line in the Declarations section if the following line is not already there:
Option Explicit
Type the following procedure:
Type the following line in the Immediate window, and then press ENTER:
? ElapsedTime(#6/1/1999 8:23:00PM#-#6/1/1999 8:12:12AM#)
Notice that the following values appear:
Compare date data
Tcl Program To Calculate The Time Difference Between Two Times Two
Because dates and times are stored together as double-precision numbers, you may receive unexpected results when you compare Date/Time data. For example, if you type the following expression in the Immediate window, you receive a False result even if today's date is 3/31/1999:
? Now()=DateValue('3/31/1999')
The Now() function returns a double-precision number that represents the current date and the current time. However, the DateValue() function returns an integer number that represents the date but not a fractional time value. Therefore, Now() equals DateValue() only when Now() returns a time of 00:00:00 (12:00:00 A.M.).

As you can see, I was able to convert seconds into days via some basic math:
- There are 60 seconds in a minute.
- There are 60 minutes in an hour.
- There are 24 hours in a day.
If you multiply the figures above, it will give you 86400, which is total the number of seconds that is in a given day. Divide the difference in seconds between our two dates by 86400 and you've got the number of days that have passed.
You can then use the floor function in order to round the result down. i.e. 2.9 days = 2 days.
To get the number of minutes, you can simply divide the difference in seconds by 60, like so:
Tcl Program To Calculate The Time Difference Between Two Times Square
2 4 6 8 10 12 14 16 18 | $then='2009-02-04'; //Convert it into a timestamp. $now=time(); //Calculate the difference. $minutes=floor($difference/60); echo$minutes; |
As you can see, it's pretty simple.
OOP
Once you've grasped the fundamentals of dealing with dates and timestamps in PHP, you should look into using the DateTime class, simply because it's cleaner and it provides you with an OO interface.
For example, if we want to get the difference in days using the DateTime class, we can do the following:
2 4 6 8 | $date2=newDateTime(); $diff=$date2->diff($date1)->format('%a'); echo$diff; |
Much better.
To transform the difference into a human-readable format that is comprised of years, months, days, hours and minutes:
2 4 6 8 10 12 14 | $then=newDateTime($then); $now=newDateTime(); $sinceThen=$then->diff($now); //Combined echo$sinceThen->m.' months have passed. '; echo$sinceThen->h.' hours have passed. '; |
As you can see, the DateTime class is pretty powerful once you figure out to use it!
Facebook Comments
Note
Office 365 ProPlus is being renamed to Microsoft 365 Apps for enterprise. For more information about this change, read this blog post.
Summary
This article describes how Microsoft Access stores the Date/Time data type. This article also describes why you may receive unexpected results when you calculate dates and times or compare dates and times.
This article describes the following topics:
- Store Date/Time data
- Format a Date/Time field
- Calculate time data
- Compare date data
- Compare time data
More Information
Store Date/Time data
Access stores the Date/Time data type as a double-precision, floating-point number up to 15 decimal places. The integer part of the double-precision number represents the date. The decimal portion represents the time.
Valid date values range from -657,434 (January 1, 100 A.D.) to 2,958,465 (December 31, 9999 A.D.). A date value of 0 represents December 30, 1899. Access stores dates before December 30, 1899 as negative numbers.
Valid time values range from .0 (00:00:00) to .99999 (23:59:59). The numeric value represents a fraction of one day. You can convert the numeric value to hours, to minutes, and to seconds by multiplying the numeric value by 24.
The following table shows how Access stores Date/Time values:
Double number | Date portion | Actual date | Time portion | Actual time |
---|---|---|---|---|
1.0 | 1 | December 31, 1899 | .0 | 12:00:00 A.M. |
2.5 | 2 | January 1, 1900 | .5 | 12:00:00 P.M. |
27468.96875 | 27468 | March 15, 1975 | .96875 | 11:15:00 P.M. |
36836.125 | 36836 | November 6, 2000 | .125 | 3:00:00 A.M. |
To view how Access stores Date/Time values as numbers, type the following commands in the Immediate window, press ENTER, and then notice the results:
?CDbl(#5/18/1999 14:00:00#)
Result equals: 36298.5833333333
?CDbl(#12/14/1849 17:32:00#)
Result equals: -18278.7305555556
To view the date and the time of numeric values, type the following commands in the Immediate window, press ENTER, and then notice the results:
?CVDate(1.375)
Result equals: 12/31/1899 9:00:00 AM Old time slot machines.
?CVDate(-304398.575)
Result equals: 8/1/1066 1:48:00 PM
Format a Date/Time field
You can format a Date/Time value to display a date, a time, or both. When you use a date-only format, Access stores a value of 0 for the time portion. When you use a time-only format, Access stores a value of 0 for the date portion.
The following table shows how Access stores Date/Time values. The following table also shows how you can display those values by using different formats:
|Stored value (double number)| Default format (General Date)| Custom format(mm/dd/yyyy hh:nn:ss A.M./P.M.)||-------------------|---------------------|-----------------------------||36295.0| 5/15/99| 05/15/1999 12:00:00 AM||0.546527777777778| 1:07 PM| 12/30/1899 01:07:00 PM||36232.9375| 3/13/99| 10:30PM 03/13/1999 10:30:00 PM|
Tcl Program To Calculate The Time Difference Between Two Times Crossword
Note How to open a .package file. The default format for a Date/Time value is General Date. If a value is date-only, no time appears. If the value is time-only, no date appears.
Calculate time data
Because a time value is stored as a fraction of a 24-hour day, you may receive incorrect formatting results when you calculate time intervals greater than 24 hours. To work around this behavior, you can create a user-defined function to make sure that time intervals are formatted correctly.
Microsoft provides programming examples for illustration only, without warranty either expressed or implied. This includes, but is not limited to, the implied warranties of merchantability or fitness for a particular purpose. This article assumes that you are familiar with the programming language that is being demonstrated and with the tools that are used to create and to debug procedures. Microsoft support engineers can help explain the functionality of a particular procedure, but they will not modify these examples to provide added functionality or construct procedures to meet your specific requirements. To correctly calculate and to format time intervals, follow these steps:
Create a module, and then type the following line in the Declarations section if the following line is not already there:
Option Explicit
Type the following procedure:
Type the following line in the Immediate window, and then press ENTER:
? ElapsedTime(#6/1/1999 8:23:00PM#-#6/1/1999 8:12:12AM#)
Notice that the following values appear:
Compare date data
Tcl Program To Calculate The Time Difference Between Two Times Two
Because dates and times are stored together as double-precision numbers, you may receive unexpected results when you compare Date/Time data. For example, if you type the following expression in the Immediate window, you receive a False result even if today's date is 3/31/1999:
? Now()=DateValue('3/31/1999')
The Now() function returns a double-precision number that represents the current date and the current time. However, the DateValue() function returns an integer number that represents the date but not a fractional time value. Therefore, Now() equals DateValue() only when Now() returns a time of 00:00:00 (12:00:00 A.M.).
To receive accurate results when you compare date values, use one of the following functions. To test each function, type the function in the Immediate window, substitute the current date for 3/31/1999, and then press ENTER:
To return an integer value, use the Date() function:
?Date()=DateValue('3/31/1999')
To remove the fractional part of the Now() function, use the Int() function:
?Int(Now())=DateValue('3/31/1999')
Compare time data
When you compare time values, you may receive inconsistent results because a time value is stored as the fractional part of a double-precision, floating-point number. For example, if you type the following expression in the Immediate window, you receive a false (0) result even though the two time values look the same:
var1 = #2:01:00 PM#
var2 = DateAdd('n', 10, var1)
? var2 = #2:11:00 PM#
When Access converts a time value to a fraction, the calculated result may not be identical to the time value. The small difference caused by the calculation is sufficient to produce a false (0) result when you compare a stored value to a constant value.
To receive accurate results when you compare time values, use one of the following methods. Games with dice. To test each method, type each method in the Immediate window, and then press ENTER:
Add an associated date to the time comparison:
var1 = #1/1/99 2:01:00 PM#
var2 = DateAdd('n', 10, var1)
? var2 = #1/1/99 2:11:00 PM#
Convert the time values to string data types before you compare them:
var1 = #2:01:00 PM# Dancing drums slot machine app.
var2 = DateAdd('n', 10, var1)
? CStr(var2) = CStr(#2:11:00 PM#)
Use the DateDiff() function to compare precise units such as seconds:
var1 = #2:01:00 PM# Serial bento 4.1.2 mac.
var2 = DateAdd('n', 10, var1)
? DateDiff('s', var2, #2:11:00 PM#) = 0
References
For more information about calculating date values and time values, see DateSerial Function
For more information about how to format Date/Time data types, click Microsoft Access Help on theHelp menu, type format property - date/time data type in the Office Assistant or the Answer Wizard, and then click Search to view the topic.